Coursework - Year 1
Assignment 1 - Tax Chart and Calculator
The first coursework we were tasked with in Java involved creating a Tax Calculator which then displayed a bar chart showing income and amount to pay.
Show me the code!
/**
* Prints table of data with gross,tax paid and net values
*
* @param gross gross income before tax
*/
public void printTable(int[]gross){
int[] net;
net = new int[gross.length];
//for loop prints each of gross income, net income , and tax paid on the gross income.
for(int row = 0;row<=(gross.length -1);row++){
net[row] = ((gross[row])-(TaxCalculator.taxPayable(gross[row])));
//ensures the net income is never less then 0
if (net[row]<0){
net[row]=0;
}
//prints out the values to the console
System.out.println("Gross:" +gross[row] + " Tax-Payable:" + (TaxCalculator.taxPayable(gross[row])) + " Net:" + net[row]);
}
}
Assignment 2 - Hotel booking system
The second coursework we were tasked with in Java involved creating a hotel booking system using OOP; this did not require a GUI.
Assignment 1 - Web Browser
The coursework we were tasked with in Java in the second semester was to create a browser that would display webpages and required a GUI, we were told to use Swing for this.
Assignment 1 - Programming Presentation
Research and present information on a programming language of our choosing, including a written report of the information.
Assignment 2 - Ethics in Software Engineering
This coursework involved choosing a computing disaster to discuss, mainly about the ethics behind the disaster, what happened, how it happened and what could have been done to prevent it.
The topic I decided to choose was 000webhost and their database breach of plain text information; over 13.5 million users details and passwords.
Assignment 3 - Usability Analysis (Sprint)
Using Nielsen's 10 Usablity Heuristics where appropriate we were tasked with testing out the universities blackboard system.
Assignment 4 - Literature Review
Using Google Scholar we were to find 3 literature pieces from trusted and reliable sources based on a subject in computing science, I opted to choose AI and Machine Learning; this wasn't a suggested topic however I find this subject interesting.
Assignment 5 - Debugging PreWritten Code
The code we were given had been written specifically for the purpose of testing and debugging, it had issues injected purposefully.
Assignment 1 - Lift Simulator
Using assembly language, we were tasked with controlling a lift.
We had a very limited amount of memory to work with.
Show me the code!
; ----- LIFT --------------------------
JMP Start ; Skip the db's
db A8 ; Code for Timer Interupt stored in A8
db 00 ; Keyboard Interupt not used
db AA ; Keypad Interupt Code stored in AA
Start:
STI ; Set the Interupt
MOV CL, 4F ;o ; Set Register CL to char o
MOV DL, 54 ;t ; Set Register DL to char t
OUT 08 ; Display Key Pad
OUT 06 ; Display lift window
JMP WaitUpButton ; Jump to check for button pressed
;------------ Display up and start up motor ------------
UP:
IN 06 ; Read Lift Status
AND AL, 04 ; Isolate Near top check bit
JNZ WaitDownButton ; If near top go back to wait for buttons
; else continue
MOV BL, 55 ; Display "UP"
MOV [C0], BL ; |
MOV BL, 50 ; |
MOV [C1], BL ; v
MOV BL, 0 ; Clear Excess Letters using null
MOV [C2], BL ; |
MOV [C3], BL ; |
MOV [C4], BL ; |
MOV [C5], BL ; v
OR AL, 01 ; Set UP motor bit
OUT 06 ; Turn on UP motor
;------------ Start checking if near top -----------------
CheckU:
AND AL, 4 ; Isolate near top check bit
IN 06 ; Read lift status
JZ CheckU ; If reached top continue else keep checking
AND AL, FE ; Turn off up motor
OUT 06 ; Send command to lift - turns off lift motor
MOV [C0], DL ; T
MOV [C1], CL ; O
MOV BL, 50 ; set bl to p
MOV [C2], BL ; P
;------------- Check if any buttons have been pressed -----------
WaitDownButton:
IN 06 ; Read lift status
AND AL, 10 ; Isolate down button
JNZ Down ; Jump to down if pressed else continue
JMP WaitDownButton ; Keep checking
WaitUpButton:
IN 06 ; Read Status again
AND AL, 20 ; Isolate up button
JNZ UP ; Jump to up if Pressed else continue
JMP WaitUpButton ; Keep checking till button pressed
;------------ Display Down and start down motor ----------------
Down:
IN 06 ; Read lift status
AND AL, 08 ; Isolate near bottom check bit
JNZ WaitUpButton ; If near bottom, go back and wait for buttons
; Else continue
MOV BL, 44 ; BL set to D
MOV [C0], BL ; D
MOV [C1], CL ; O
MOV BL, 57 ; BL set to W
MOV [C2], BL ; W
MOV BL, 4E ; BL set to N
MOV [C3], BL ; N
OR AL, 02 ; Set Down motor bit
OUT 06 ; Turn on Down motor
;------------ Start checking if lift is at bottom --------------
CheckD:
AND AL, 08 ; Isolate near bottom check bit
IN 06 ; Read lift status
JZ CheckD ; If near bottom continue else keep checking
AND AL, FD ; Turn off down motor
OUT 06 ; Send command to lift - turns off lift motor
MOV BL, 42 ; Set BL to B
MOV [C0], BL ; B
MOV [C2], DL ; T miss O as this would already be there from dOwn
MOV [C3], DL ; T
MOV [C4], CL ; O
MOV BL, 4D ; Set Bl to M
MOV [C5], BL ; M
JMP WaitUpButton ; Go back to wait for buttons again
ORG A8
iret ; Do nothing, return
ORG AA
POP AL ; POP to prevent code overwriting, done first to prevent
; quick presses of button affecting code
IN 08 ; Port for the peripheral keypad
CMP AL,0D ; Check if enter was pressed on pad
JZ DOWN ; If it was jump to Down
IN 06 ; Read Lift Status
AND AL, 1 ; Check if up motor was on
JNZ CheckU ; If it was check if near top to prevent crash
JMP CheckD ; Else check if near bottom to prevent crash
END
; --------------------------------------------------------------
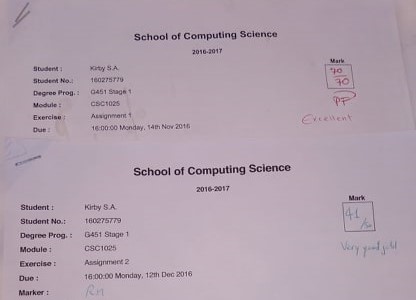
Assignment 1 - Fundamental Maths
Some of the topics we covered for this coursework included.
- Basics of Set Theory
- Numbers and their representations
- Real Valued Functions
- Vectors and Matrices
- Combinatorics and Basics of Counting
Assignment 2 - Discrete Maths
Some of the topics we covered for this coursework included.
- Relations and Functions
- Proof Techniques
- Recursive Function Definitions and Proof by Induction
- Graph Theory
Assignment 1 - Copy a Website using image
Using just an image and some pre-written text, we were tasked with marking up the text so that the page had the same underlying structure as a website and then using CSS to make it look like the final result.
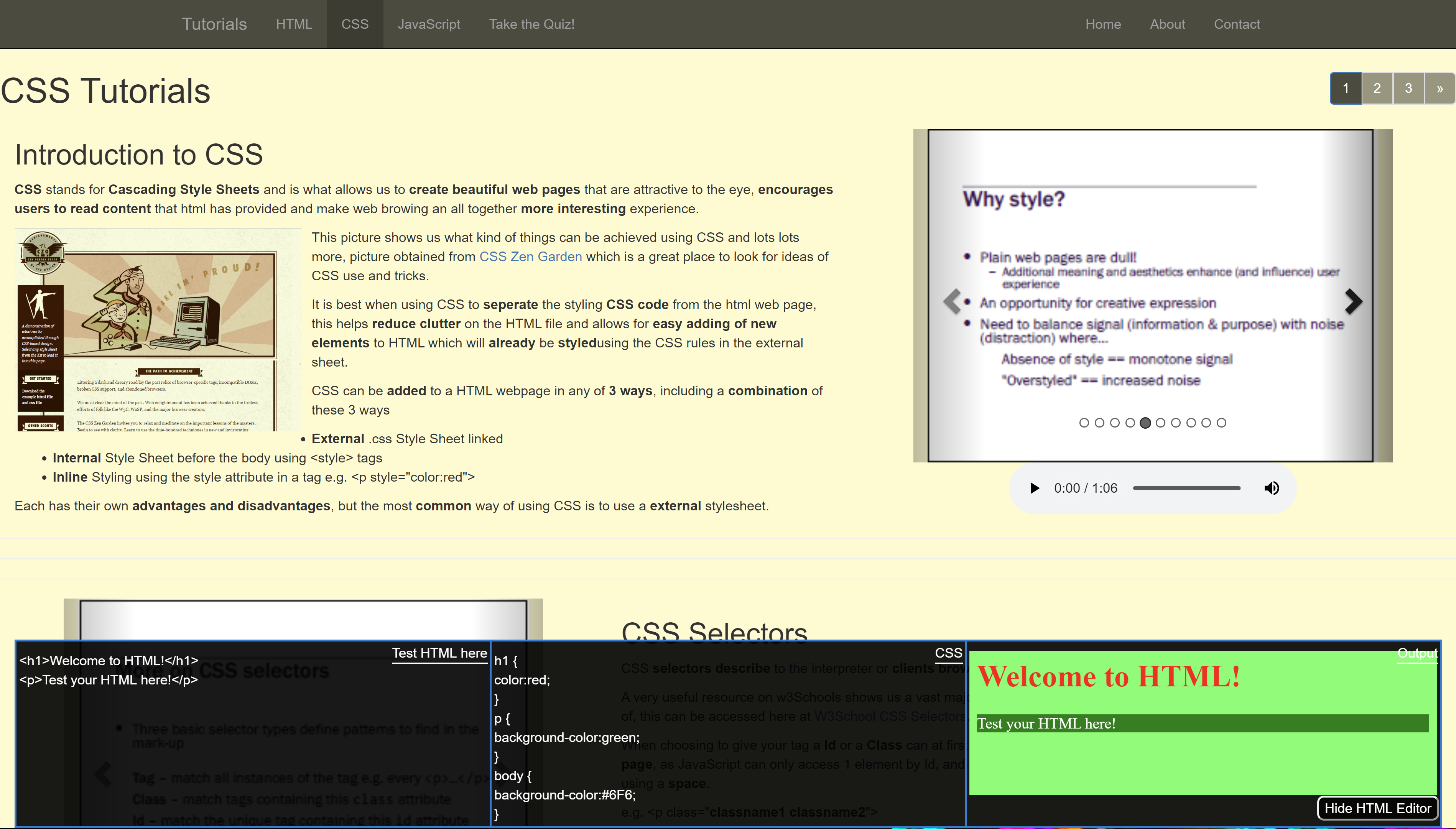
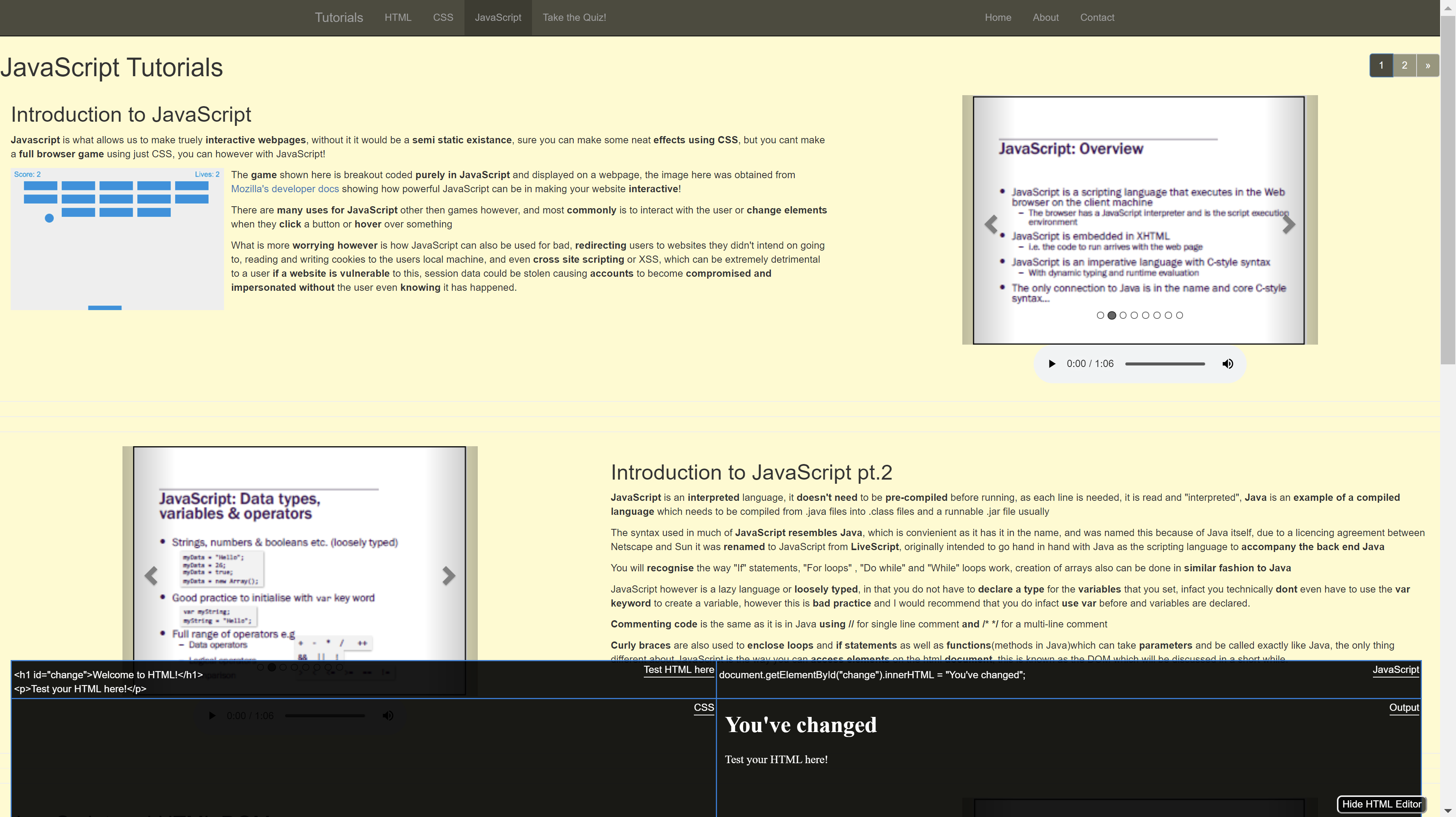
Assignment 2 - Develop Tutorial Website
Voice clips and slides from the course are laid out in easy to use sections separating the different topics of the course.
The user can utilise what they have learnt from the website and gain feedback immediately by using the live HTML, CSS, Javascript testing area, this function was adapted from some code I found online, the layout changes depending on what section of the tutorial website the user is on (shown in the pictures).
This testing area can expand to accommodate more lines as needed.
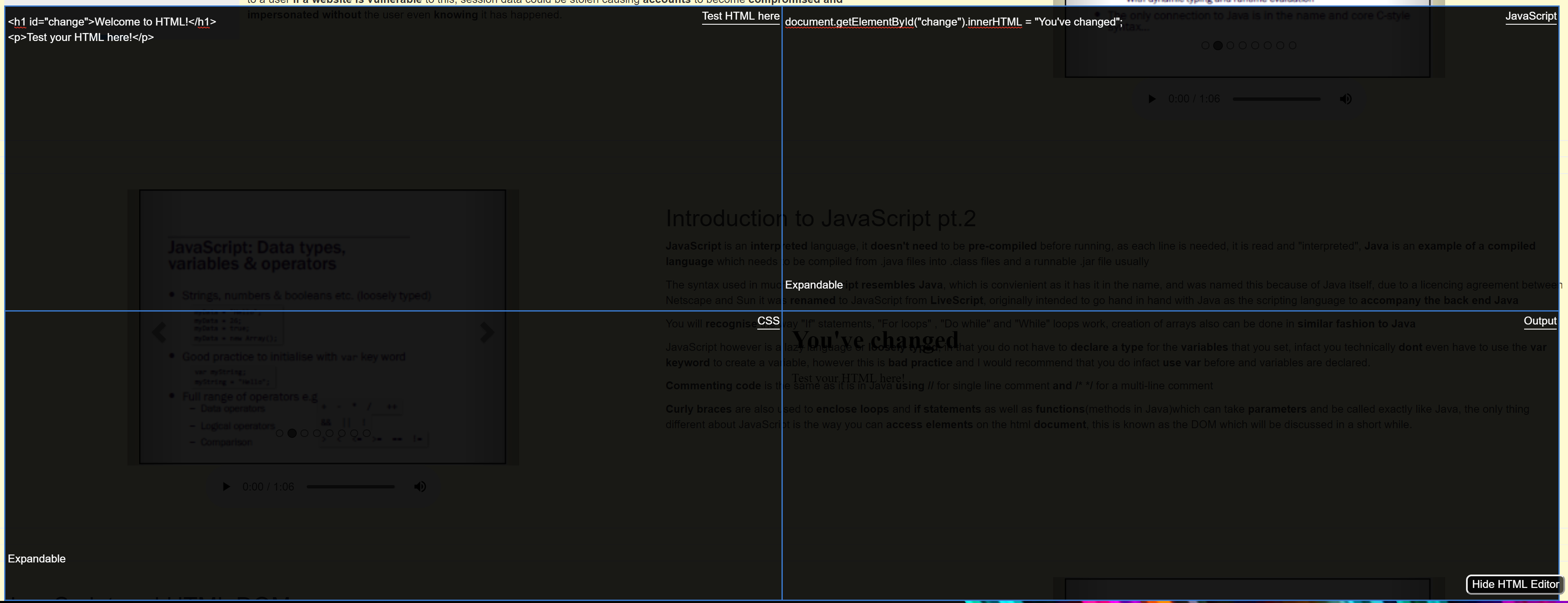